
Why is this bad ?
After all we all want to get our hands on the latest RTX graphic cards, and run our games at highest possible frame rate (after enabling all the detailed rendering options).
Faster is Better, unfortunately, is not always the case when you are programming a game object to move.
By inheriting from UnityEngine’s MonoBehaviour, we can move our object in the Update() method:
using UnityEngine;
public class Player : MonoBehaviour
{
// Update is called once per frame
void Update()
{
//code to move our game object
}
}
The problem is that, with your game running 60 frames per seconds (or higher), any movement code inside Update() will be running 60 times within a second.
Now imagine you want a background game object rotates roughly at 6 degree per second. A full 360 degree rotation will then take 1 min to complete.
If you just put that “moving 6-degrees-per-second logic” inside Update(), then it will become “moving 6-degrees-per-frame”, which means that with 60 frames/second runtime, a full 360 degree rotation takes only 6 seconds to complete. Too fast !
using UnityEngine;
public class Player : MonoBehaviour
{
void Start()
{
}
// Update is called once per frame
void Update()
{
transform.Rotate(new Vector3(0, 6, 0));
}
}
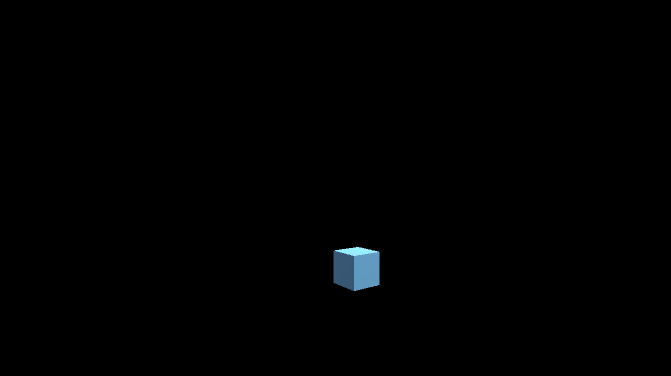
How to fix this ?
To move at normal real-time speed (of roughly a second), simply multiply “Time.deltaTime” like this:
using UnityEngine;
public class Player : MonoBehaviour
{
void Start()
{
}
// Update is called once per frame
void Update()
{
transform.Rotate(new Vector3(0, 6, 0) * Time.deltaTime);
}
}

Happy controlling your speed !